Mastering Python for Automation and Scripting: A Comprehensive Guide
Python is one of the most versatile and widely-used programming languages in the world,
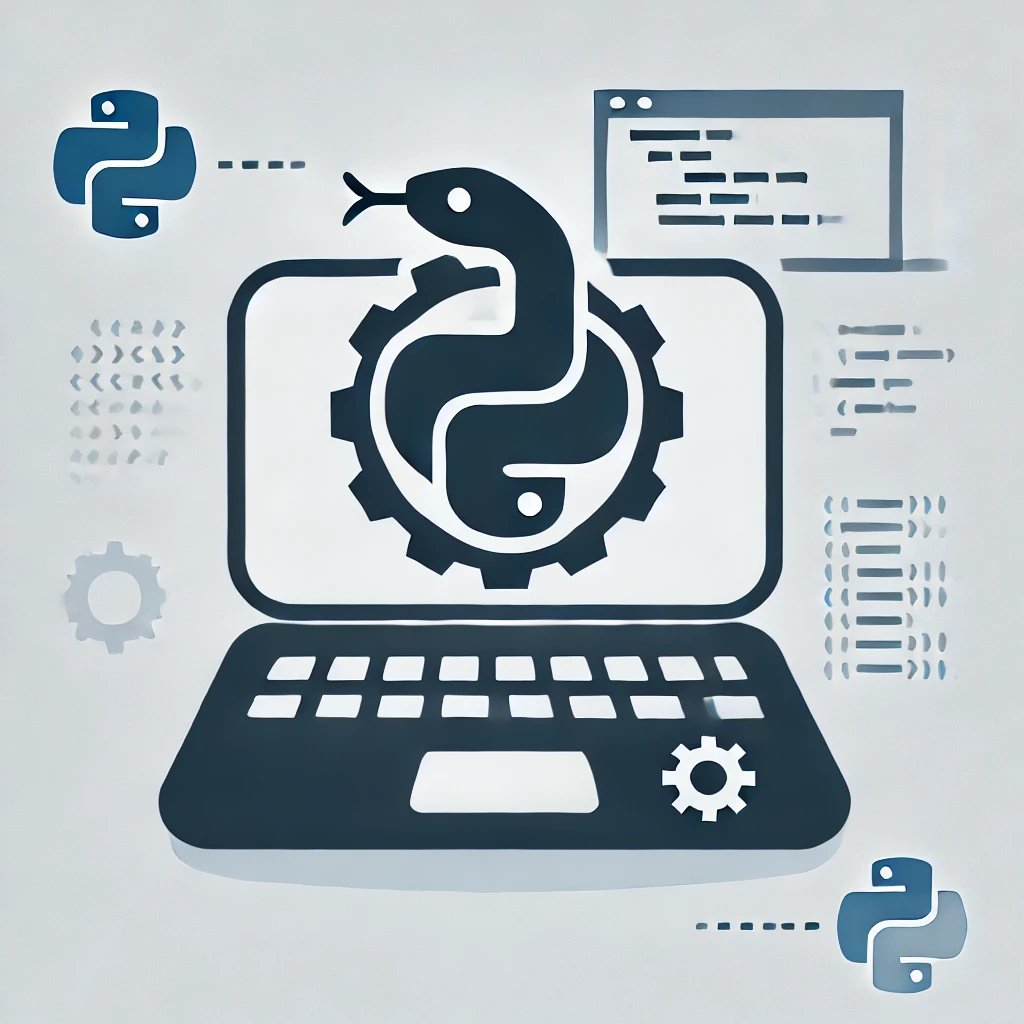
Python is one of the most versatile and widely-used programming languages in the world, especially for automation and scripting tasks. Its simplicity, flexibility, and rich ecosystem of libraries make it an essential skill for anyone in tech, including system administrators, developers, cybersecurity professionals, and data analysts. This article will guide you through mastering Python for automation and scripting.
Why Python for Automation?
-
Simplicity: Python’s easy-to-read syntax makes it accessible for beginners.
-
Versatility: It can handle everything from file manipulation to web scraping and network automation.
-
Large Library Support: Python’s extensive library ecosystem (e.g., os, shutil, requests, selenium) provides tools for virtually any automation task.
-
Cross-Platform: Python works seamlessly on Windows, Linux, and macOS.
Key Areas to Focus On
Master Python Basics
Before diving into automation, ensure you’re comfortable with:
Variables and Data Types:
name = "Alice"
age = 25
print(f"{name} is {age} years old.")
Control Structures:
for i in range(5):
print(i)
Functions:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
File Handling:
with open("example.txt", "w") as file:
file.write("This is a sample file.")
Automating System Tasks
File and Directory Management: Automate file operations using the os and shutil modules:
import os, shutil
os.mkdir("new_folder")
shutil.copy("source.txt", "new_folder/destination.txt")
Scheduling Scripts: Use schedule or cron (Linux) to run Python scripts automatically.
import schedule, time
def job():
print("Task running...")
schedule.every(10).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
Web Automation
Web Scraping: Extract data from websites using libraries like BeautifulSoup and requests:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
print(soup.title.text)
Browser Automation: Automate browsers using selenium:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com")
driver.quit()
Network Automation
Automate network tasks like SSH connections or device configurations:
Using paramiko for SSH:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect("192.168.1.1", username="admin", password="password")
stdin, stdout, stderr = ssh.exec_command("ls")
print(stdout.read().decode())
ssh.close()
Automating Emails
Send automated emails using the smtplib library:
import smtplib
server = smtplib.SMTP("smtp.gmail.com", 587)
server.starttls()
server.login("your_email@gmail.com", "password")
server.sendmail("your_email@gmail.com", "recipient@gmail.com", "Hello, this is an automated email.")
server.quit()
Data Automation
Automate data collection, cleaning, and reporting:
Use pandas for data manipulation:
import pandas as pd
data = {"Name": ["Alice", "Bob"], "Age": [25, 30]}
df = pd.DataFrame(data)
df.to_csv("output.csv", index=False)
Real-World Use Cases
-
System Administration: Automate tasks like backups, log monitoring, and user account management.
-
Cybersecurity: Create scripts for vulnerability scanning, log analysis, or brute force simulations.
-
Web Development: Automate testing, deployment, and server configurations.
-
Data Analysis: Automate data scraping, cleaning, and visualization for reports.
-
Tools and Libraries for Automation
-
File Operations: os, shutil
-
Web Scraping: requests, BeautifulSoup, Scrapy
-
Browser Automation: selenium
-
Network Automation: paramiko, netmiko
-
Task Scheduling: schedule, apscheduler
-
Data Handling: pandas, openpyxl
Best Practices
-
Write Modular Code: Break scripts into reusable functions.
-
Error Handling: Use try...except to handle unexpected issues.
try:
# risky operation
except Exception as e:
print(f"Error: {e}")
-
Document Your Code: Use comments and docstrings for clarity.
-
Test Regularly: Test scripts in different environments to ensure reliability.
Conclusion
Python is an indispensable tool for automation and scripting. By mastering Python, you can save time, boost productivity, and tackle complex tasks efficiently. Whether you’re a system admin, developer, or cybersecurity professional, Python automation can transform the way you work. Start small, build projects, and keep expanding your knowledge to become an expert in Python automation.
Leave a Comment
Releted Articles
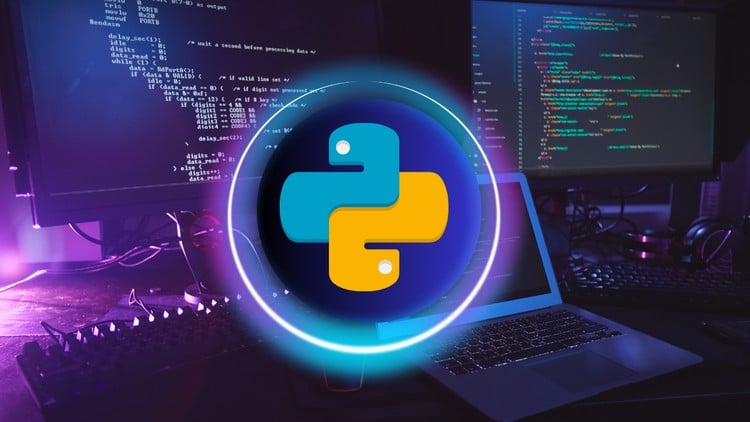
Python Programming for Beginners: Learn Python from Scratch – Course Review
This course is designed to take beginners from zero to proficiency in Python
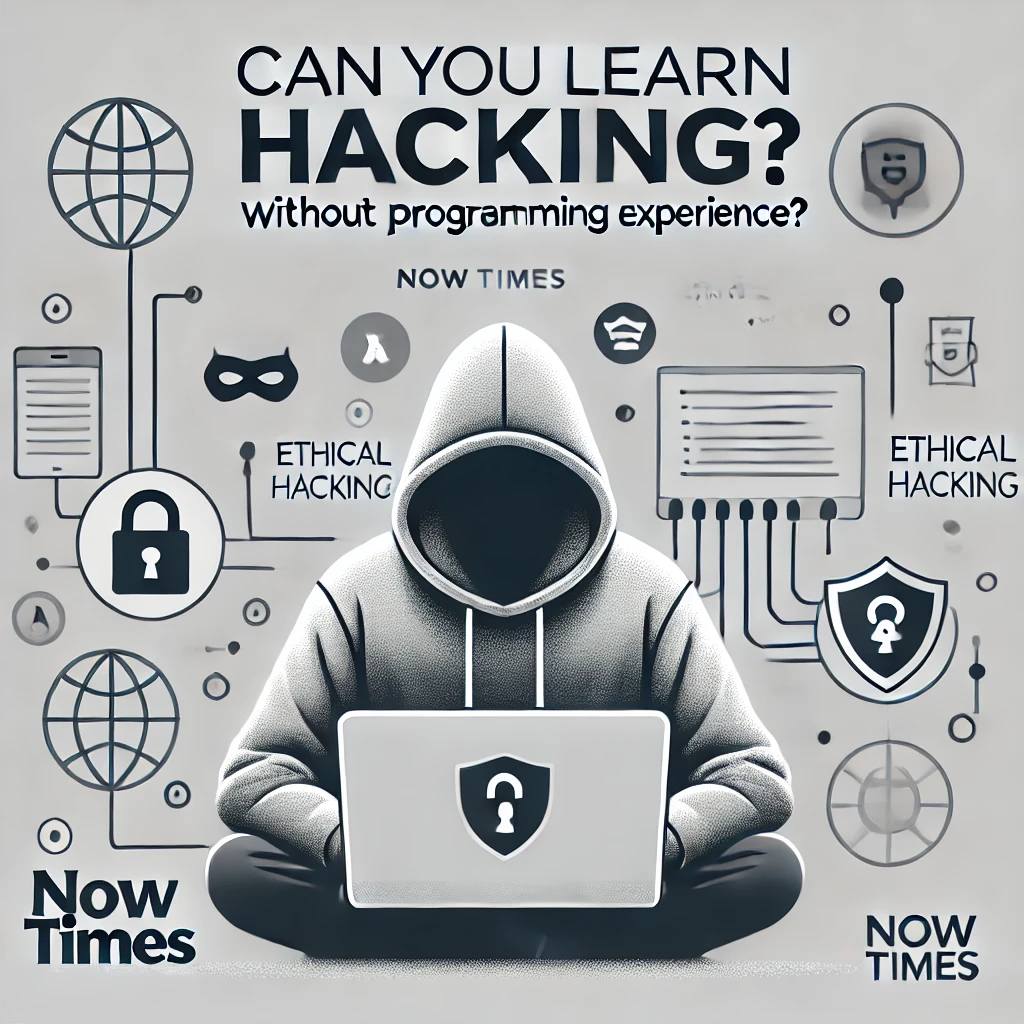
Can You Learn Hacking Without Programming Experience? A Complete Guide
While programming is a valuable skill in ethical hacking, it is not a strict requirement for getting started.
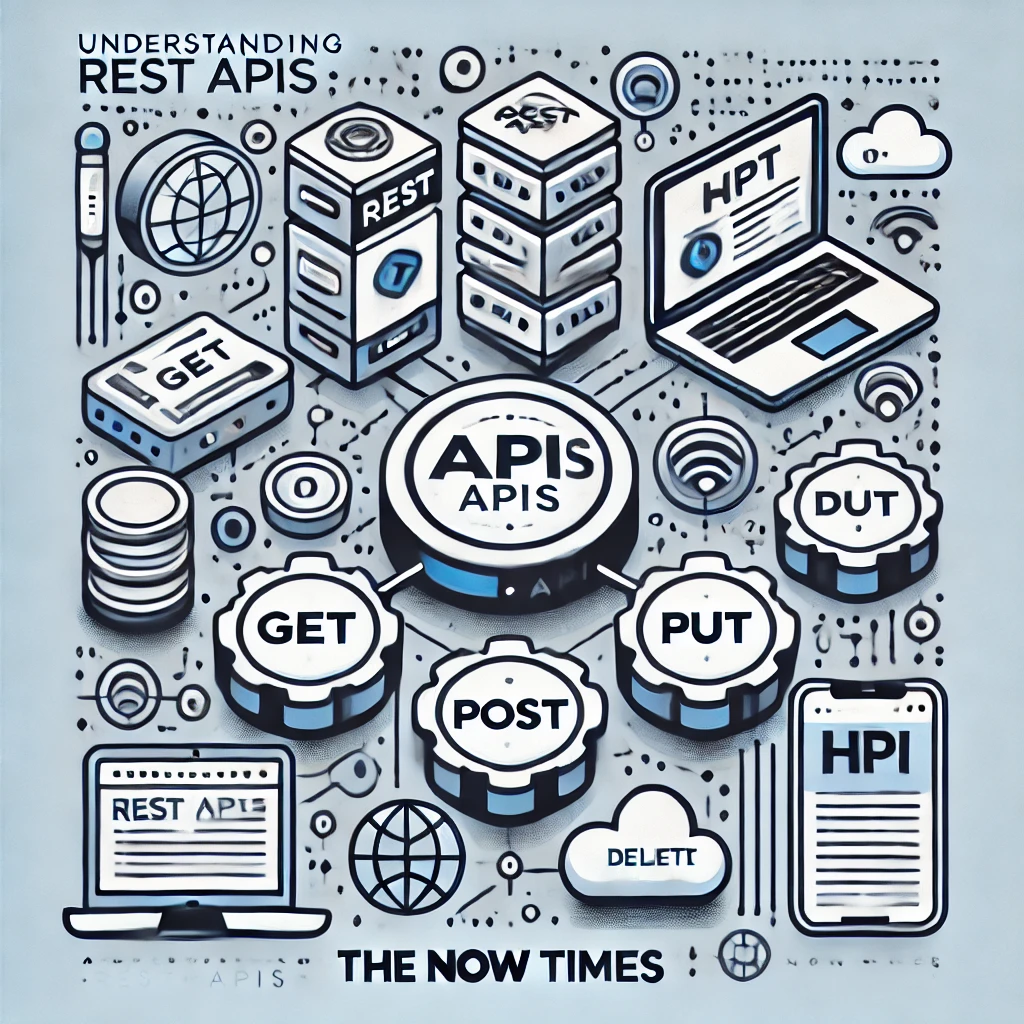
Understanding REST APIs: A Continuation of API and Their Types
REST APIs have revolutionized the way systems communicate over the web. Their simplicity, scalability, and compatibility with standard web protocols m
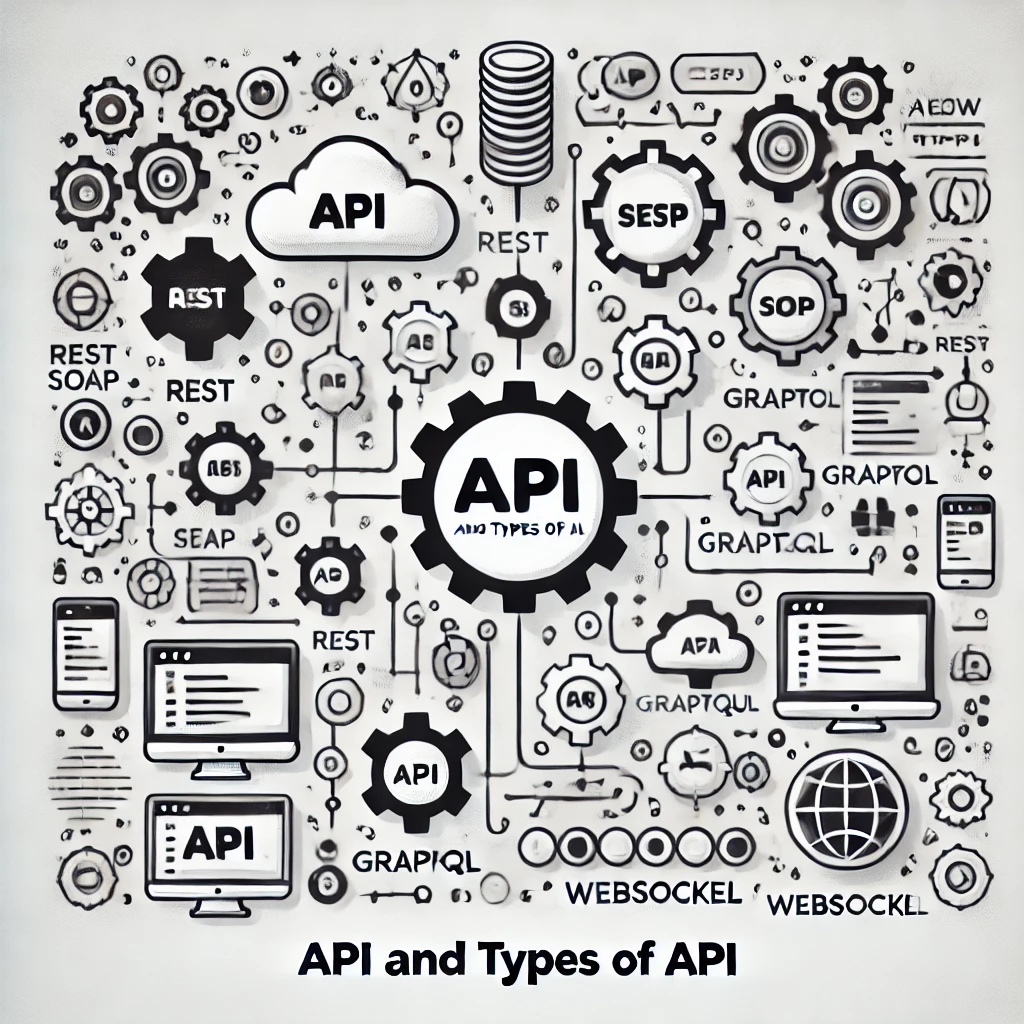
Understanding APIs and Their Types
APIs are the building blocks of modern software development, enabling seamless communication between diverse systems.
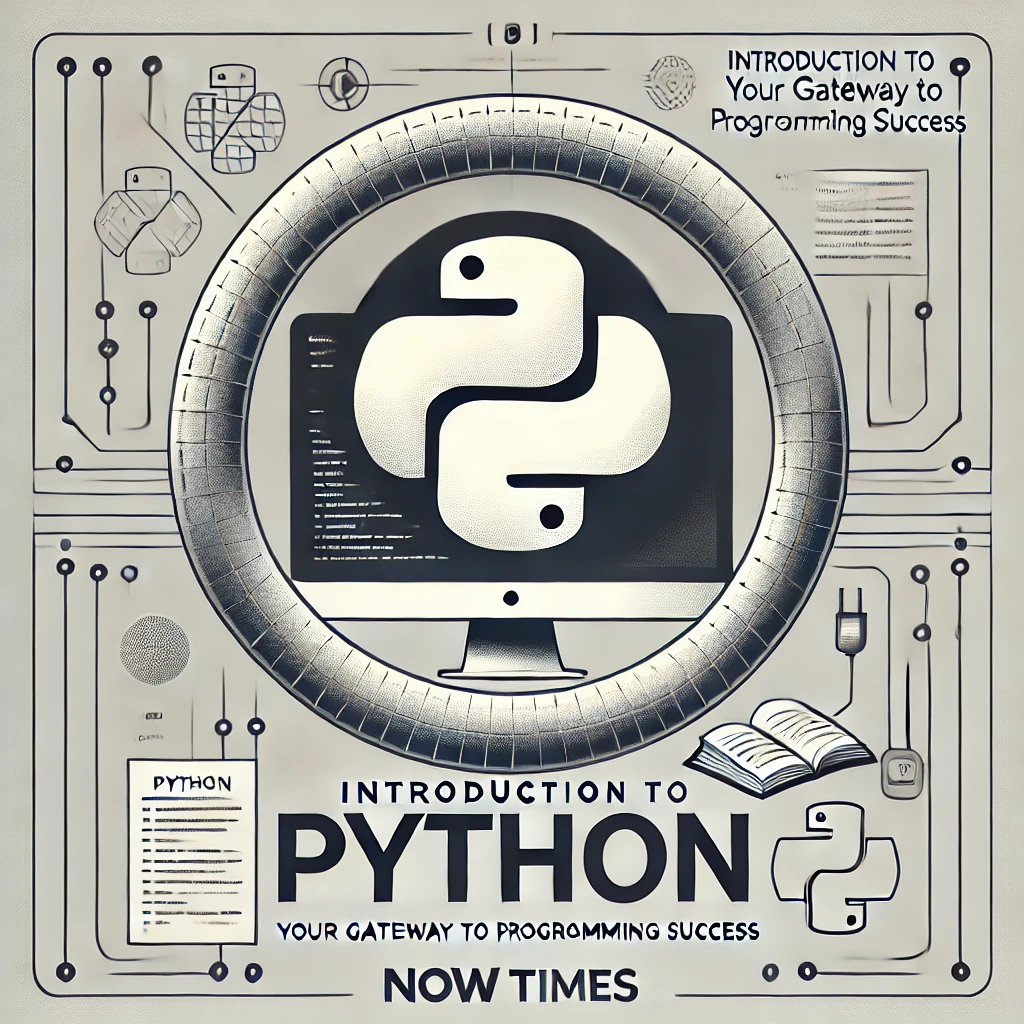
Introduction to Python: Your Gateway to Programming Success
Python is widely used in various fields, making it an invaluable skill
1 comments
CyberSmart
Feb. 1, 20250 replies CyberSmart